ESLint
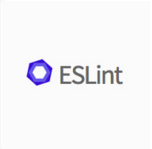
Language / Framework: JavaScript
Checks: 190+
Categories: Complexity, Style
Channels: stable
: ESLint v3.19.0, eslint-1
: ESLint v1.10.3, eslint-2
: ESLint v2.10.2, eslint-4
: ESLint v4.15.0, eslint-5
: ESLint v5.7.0, eslint-6
: ESLint v6.1.0, eslint-7
: ESLint v7.6.0, eslint-8
: ESLint v8.36.0
ESLint offers linting, complexity analysis, and style checking for your modern EcmaScript/JavaScript code.
Enable the Plugin
To enable ESLint analysis, add the following to your .codeclimate.yml configuration file:
plugins:
eslint:
enabled: true
More information about the CLI is available in the README here: https://github.com/codeclimate/codeclimate
ESLint Versions
To use ESLint versions for your analysis, specify a channel within your .codeclimate.yml configuration file:
plugins:
eslint:
enabled: true
channel: "eslint-8"
plugins:
eslint:
enabled: true
channel: "eslint-7"
plugins:
eslint:
enabled: true
channel: "eslint-6"
Note:
If no channel is specified, ESLint v3.19.0 is used for analysis.
Configure the Plugin
ESLint requires configuration via a .eslintrc
configuration file as detailed in ESLint's documentation.
Adding a .eslintrc.js
You'll need to add a .eslintrc.js (or .eslintrc) config file to your project if it doesn't have one already.
If you have your eslint configuration saved to another file in your repo, you can configure the engine to use that path instead of the default path:
eslint:
enabled: true
config:
config: path/to/.eslintrc
It is important to note that a .eslintrc.js config file is typically required for our ESLint engine to analyze your code.
Extensions
You can configure our ESLint Engine to analyze the file types you’d like by configuring the extensions in your .codeclimate.yml
, as shown in the example below:
eslint:
enabled: true
config:
extensions:
- .es6
- .js
- .jsx
- .mjs
- .vue
By default, the ESLint engine only analyzes
.js
files. If you want ESLint to analyze your JSX code and ES6 files, you'll need to specify this in your.codeclimate.yml
, as shown in the example above.
Customizing Checks and Thresholds
Users can adjust their ESLint configuration through a variety of means, including:
- Directly disable a check in their
.codeclimate.yml
file:
plugins:
eslint:
enabled: true
checks:
complexity:
enabled: false
- Enable and disable rules in your .eslintrc with 0 (disabled), or 2 (enabled - and issue reported as error, required for Code Climate engine to treat it as an issue).
- Configure tolerance for violations (including complexity) by tuning the threshold in their .eslintrc under the Rule name. For instance:
{
"env": {
"node": true
},
"rules": {
"brace-style": [2, "1tbs", { "allowSingleLine": true }],
"comma-dangle": [2, "never"],
"comma-style": [2, "first", { exceptions: {ArrayExpression: true, ObjectExpression: true} }],
"complexity": [2, 6],
"curly": 2,
"eqeqeq": [2, "allow-null"],
"max-statements": [2, 30],
"no-shadow-restricted-names": 2,
"no-undef": 2,
"no-use-before-define": 2,
"radix": 2,
"semi": 2,
"space-infix-ops": 2,
"strict": 0
},
"globals": {
"AnalysisView": true,
"PollingView": true,
"Prism": true,
"Spinner": true,
"Timer": true,
"moment": true
}
}
Users may also exclude a particular issue found by excluding its fingerprint.
plugins:
eslint:
enabled: true
exclude_fingerprints:
- c1602d46125c5d6c015c56d6bc5f6b9d
Rules can also be marked at the warning level (1). By default, these will be registered as an error. They can be ignored using the ignore_warnings flag.
plugins:
eslint:
enabled: true
config:
ignore_warnings: true
Understand the Plugin
A full list of available checks can be found here. Edit your .eslintrc
to select rules and tune thresholds that best meet the requirements of your team.
Supported Plugins
For security concerns related to executing code within a docker container, our ESLint engine currently ignores all but a select number of ESLint plugins.
To view the supported plugins for each of our ESLint channels, check out the links below:
eslint-8
- (here)
eslint-7
- (here)
eslint-6
- (here)
eslint-5
- (here)
eslint-4
- (here)
eslint-3
- (here)
We hope to build support for additional plugins soon.
Updated over 1 year ago